Explaining Javascript Array Methods
Differences Between Filter, Find, Map and ForEach Methods
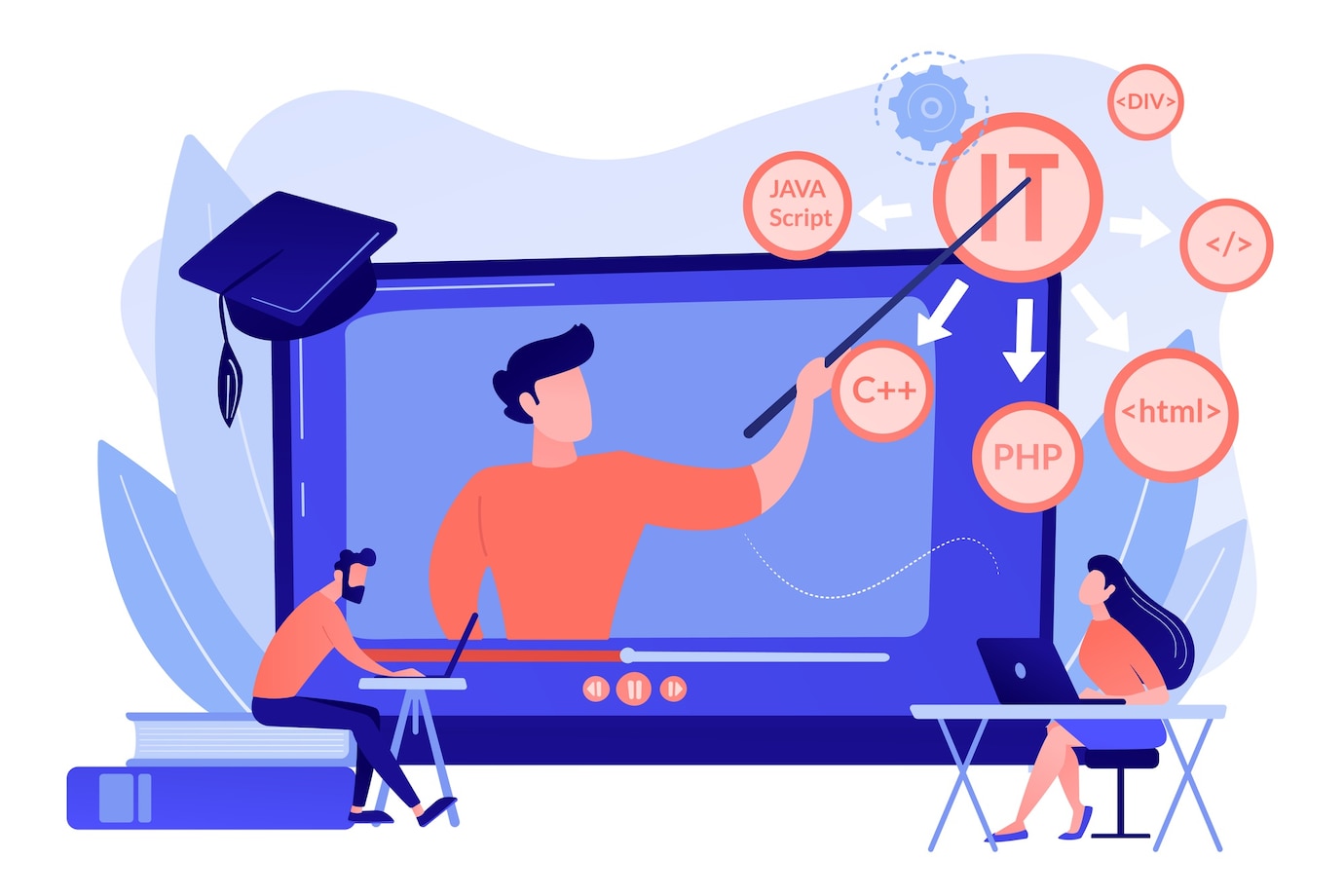
Explaining the differences of Filter, Find, Map and ForEach Methods in Javascript
Introduction
Howdy! It’s been a long time since the last post 😁. I’ve been so busy with work and after work, I don’t feel like coding or writing anything. But I’ve come back now. I have so many different ideas for posts but I’ve lost them along the way. Recently, I tutored a friend to learn Javascript and React. She told me that her friend had an interview with a company for a Developer position. The interviewer asked him if he knew the differences between the map
and forEach
array methods in Javascript. Therefore, I had an idea of writing a post explaining this topic to check my knowledge as well.
Array
In any programming languages, there will be primitive data type
and non-primitive data type
. The following examples are primitive data types:
- string
- number
- boolean
- undefined
- null
In Javascript, if a value is not an object and has no methods or properties, it’s a primitive value
. Primitives
are immutable as well which means they can’t be altered.
On the opposite, array
in Javascript is not primitive because it is resizable and has many methods. The following are characteristics of array
- can contain a mix of different data type
- are
zero-indexed
which means the first element of array is at index 0 - array-copy operations create
shallow copies
instead ofdeep copies
We will get to the shallow copies vs deep copies topic alter in another post.
Array Methods
Array data type in Javascript has so many different methods, this is one of the sources that you could check out. Today we take a look at these 4 following methods in-depth:
- Filter
- Find
- Map
- ForEach
Filter vs Find
A simple explanation for both filter
and find
method is that they both try to find elements in the array for you that fit the provided criteria. The difference is that filter
method returns a shalow copy
of the original array meanwhile find
returns the first element that satisfies the criteria.
Given this original array
|
|
What if we only want to find negative values from this array? This is when we should use filter
method because we want to find more than 1 element
|
|
The method loops through the original array and then creates its shallow copy that contains only the negative values thanks to the callback function we declare above. But what if we just want to find number 0
in the array? If so, we should use find
method instead.
|
|
As we could see here that the method returns only a single value compared to filter
. What if we try to find not existed values in the array?
|
|
In this case, we try to find value/values that is/are more than 100
. The original array doesn’t contain any of that value, therefore, returns an empty array []
for filter
and undefined
for find
.
Map vs ForEach
In short, both map
and forEach
methods loop through the original array and change each element according to the required criteria defined in the callback function. The main difference is that map
returns a shallow copy
of the original array but forEach
returns undefined
instead. Let’s take a deeper look into the following piece of code.
|
|
We can see that although both methods can loop through the original and transform its elements, forEach
returns undefined
while map
returns a copy with each value transformed into strings. So with forEach
we have to do an extra step
|
|
We have to create an empty outside of the loop then push the transformed values into that empty array and we get the transformed result.
So at this point, what are the differences between forEach
and the normal for
loop? They seem to behave the same way so why bother using forEach
? Well, that is correct but forEach
is more elegant to loop through values compared to the old for
loop. With forEach
, we have variables for each element we are looping through such as the val
variable above but with for
loop we have to address the variable as original[i]
. forEach
syntax is also more concise compared to for
loop. This post covers really well the differences, you could check it out!
Final Notes
I hope that after reading, you have a better insights on these popular array methods in JavaScript. There is still 1 mysterious array method that I haven’t mentioned and that is reduce
. I intended to include it in this post as well but it’s a very interesting and versatile method that I think I should cover it in another post.
See you in the next post! Be positive and stay productive! 😄
https://developer.mozilla.org/en-US/docs/Glossary/Primitive
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array
https://javascript.info/array-methods
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/find
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach
https://ibaslogic.com/javascript-foreach/